Service Worker Instructions
Service workers play a crucial role in delivering mobile-app-like features to websites.
They are necessary for enabling web push notifications, and Hood provides its own service worker implementation for this purpose.
However, web applications can only use one service worker at a time.
This guide explains how to integrate Ocamba's solution if your website already uses its own service worker.
Step 1. Specify the Path to Your Service Worker
There are 3 ways to define the path to your service worker file.
- Using the Web Settings Page
Navigate to Hood > Tags Manager > List of all tags and choose a specific tag.
Then, click on the Configure button in the top right corner of the
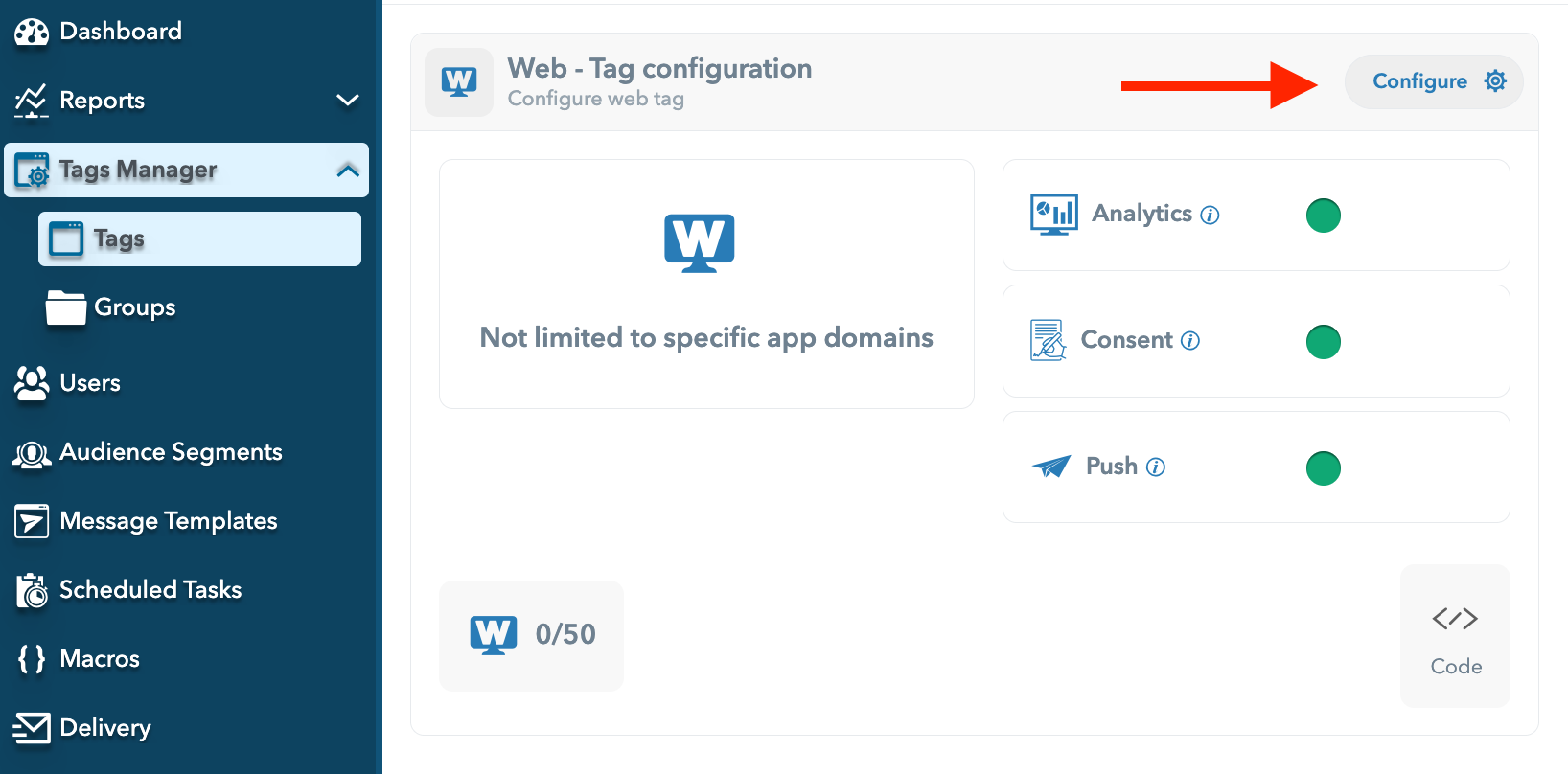
Configure Tag Button Location
Scroll down to the Push settings, and here is where you can edit the Custom Service Worker Path.
Provide the URL of your service worker.
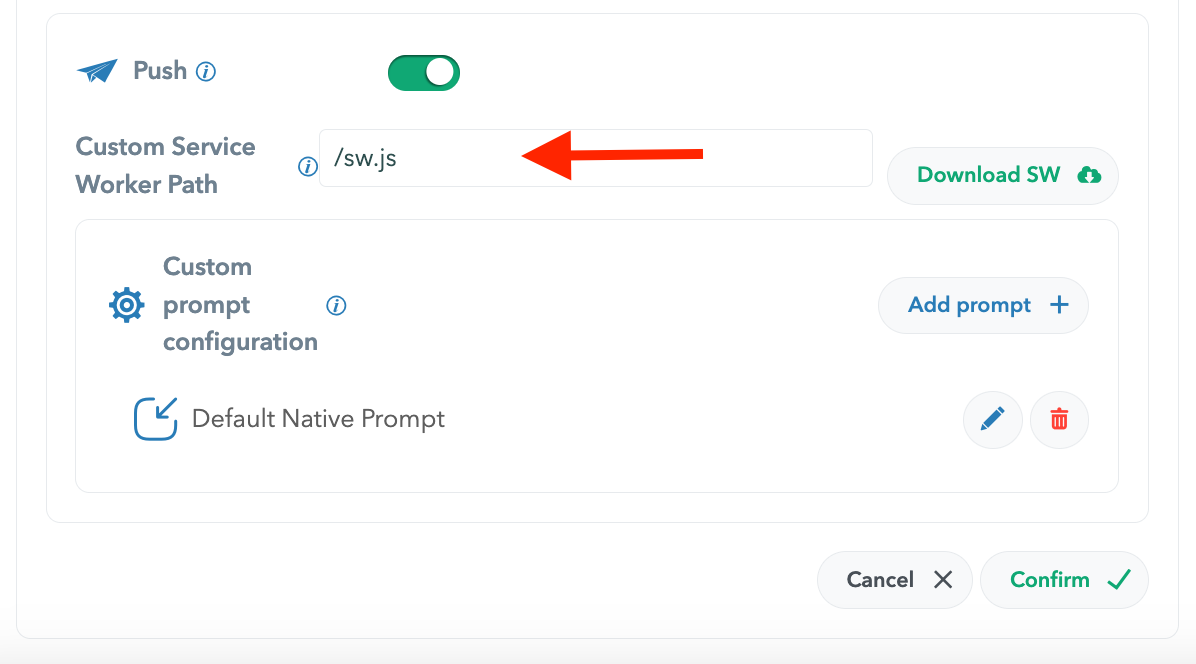
Custom Service Worker Path Location
- Through Init in Your Code
In your code, modify the initialization call as follows.
Ocamba.push(["init", {
webKey: "YOUR_WEBKEY",
serviceWorkerUrl: '/sw.js', // Adjust to the actual path of your
service worker
// … additional initialization options
}]);
Update /sw.js to reflect the correct relative path to your service worker, starting from your website's root.
- Manually Create a Service Worker
Manually create a service worker file by adding the following code inside the file:
importScripts(\"https://cdn.ocmhood.com/sdk/osw.js\");
Step 2. Incorporate the Hood Service Worker in Your File
In your existing service worker file, update the initialization code to include your YOUR_WEBKEY and add the following at the beginning of the file:
importScripts(\'https://cdn.ocamba.com/sdk/1.1/ocamba-loader.min.js\');
Ocamba = self.Ocamba \|\| \[\];
Ocamba.push(\[\'init\', {
webKey: \"YOUR_WEBKEY\",
}\]);
Replace YOUR_WEBKEY with your actual key for the Ocamba platform.
Using Workbox for Service Worker Generation
When using Workbox, add to the ‘generateSW’ step:
importScripts: \[\"./ocamba-worker-loader.min.js\"\]
Step 3. Test Your Integration
To confirm that your push notification integration is functioning correctly, subscribe to push notifications and verify their delivery.
Customizing Your Service Worker Integration
If your setup requires particular adjustments, please follow these steps:
Place service worker files into a subdirectory
Rename the files
Include multiple service workers on an origin
Options: serviceWorkerFile -> default value /sw.js serviceWorkerScope -> default value highest root folder of ServiceWorkerFile
To set up the service worker files in a subdirectory for Custom Code Setup, set up your initialization call as follows:
Hood(\'init\',\'YOUR_API_KEY\', {
push: \'YOUR_PUBLIC_PUSH_TOKEN\',
serviceWorkerFile: \'/my_sub_dir/worker.js\',
serviceWorkerScope: \'/my_sub_dir/\'
})
An alternative approach is to use the Service-Worker-Allowed HTTP Header when serving your Service Worker file. By configuring your server to return Service-Worker-Allowed: / in the response for the Service Worker, this will instruct the browser to broaden the scope and allow that scope to be used.
Default message if (oswbox){ let oswc = { title: “Your default title”, options : { “body”: “Your default body”, } }; oswbox.setConfig(oswc); }
Methods
Method | Parameters | Description |
---|---|---|
pushStatus | callback | Returns the status of a user’s notification permission in the global scope. |
pushRequestpermission | callback | Requests a permission prompt from the user for the current origin to display notifications. |
pushMessage | Notification Object,callback | Creates a web notification on an active service worker and shows it to visitors. |
Example:
function myPushStatus(status){
console.log(status);
}
Hood(‘getPushStatus’, myPushStatus)
Events
Ocamba.Hood events are accessed via event call.
Example:
Name | Listen to | Description |
---|---|---|
Push alert | onPushShow | Emitted when native push notification prompt is shown. |
Push allow | onPushAllow | Emitted when a visitor clicks on the Allow button of the notification prompt. |
Push block | onPushBlock | Emitted when a visitor clicks on the Block button of the notification prompt. |
Push blocked | onPushBlocked | Emitted when the push notification is initialized and if a returning visitor has blocked push notifications. |
Push granted | onPushGranted | Emitted when the push notification is initialized and if a returning visitor has allowed push notifications. |
Example
function myOnPushAllow(){
console.log(‘visitor allowed push’);
}
Hood(’event’,‘onPushAllow’, myOnPushAllow);
Notification Object
Name | Type | Description |
---|---|---|
title | String | The title that must be shown within the push notification. |
options | Notification Options Object | An object used to configure the notification settings. |
Notification Options Object
Name | Description |
---|---|
actions | An array of actions to be displayed in the notification. Each action of the array should be an object literal. It may include various properties, as outlined in the table below. |
action | A DOMString containing the user action to be displayed on the notification. |
title | A DOMString containing the action text to be shown to the user. |
icon | A USVString containing the URL of the icon to be displayed with the action. Appropriate responses are built using event.action within the notification click event. |
badge | A USVString containing the URL of an image to represent the notification when there is not enough space to display the notification itself such as on the Android Notification Bar. On Android devices, the badge should be designed to accommodate up to 4x resolution, approximately 96 x 96 px, and the image will be automatically masked. |
body | A string representing additional content to display within the notification. |
data | Arbitrary data that you want to be associated with the notification. This data can be of any type. |
dir | The direction of the notification; it can be auto, ltr or rtl. |
icon | A USVString containing the URL of an image to be used as an icon within the notification. |
image | A USVString containing the URL of an image to be displayed in the notification. |
lang | Specify the language used in the notification. This string must be a valid BCP 47 language tag. |
renotify | A boolean that indicates whether to suppress vibrations and audible alerts when reusing a tag value. If the renotify option is true and the tag option is an empty string, a TypeError will be thrown. The default value is false. |
requireInteraction | This setting indicates that on devices with sufficiently large screens, a notification should remain visible until the user clicks or dismisses it. If this value is not specified or set to false, the desktop version of Chrome will automatically minimize notifications after approximately 20 seconds. The default value for this setting is false. |
silent | When set, this indicates that no sounds or vibrations should occur. If the silent option is true and the vibrate option is specified, a TypeError exception will be thrown. The default value is false. |
tag | An ID for a given notification that allows you to find, replace, or remove the notification using a script if necessary. |
timestamps | A DOMTimeStamp represents the time when the notification was created. It can be used to show the actual time relevant to the notification. For example, this timestamp could be set in the past for a message that couldn’t be delivered immediately because the device was offline, or it could be set in the future for a meeting that is about to start. |
vibrate | A vibration pattern associated with the notification can be defined using an array with as few as one member. The values in the array represent times in milliseconds, where even indices (0, 2, 4, etc.) specify the duration of the vibration, and odd indices specify the duration of the pause. For example, the pattern [300, 100, 400] would cause the device to vibrate for 300 milliseconds, pause for 100 milliseconds, and then vibrate again for 400 milliseconds. |